Room이 좋다는 것을 알기 전에, SQlite가 어떤 식으로 동작하는지는 알아야, Room과 비교할 수 있겟죠?
간단하게 SQLite을 어떻게 쓰는지 코드로 알아보겠습니다.
전체적인 느낌은 DB에 SQL문을 날릴 때의 느낌과 유사합니다. 간단하게 INSERT/GETALL/DELETE 정도만 구현해봤습니다.
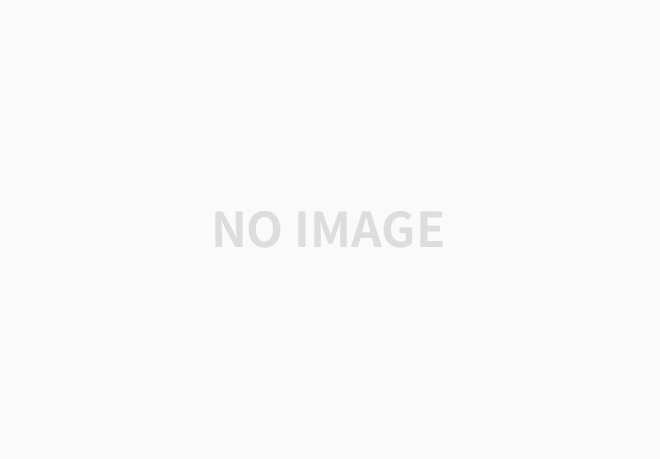
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainActivity : AppCompatActivity() { | |
lateinit var db : SQLiteHelperSample | |
override fun onCreate(savedInstanceState: Bundle?) { | |
super.onCreate(savedInstanceState) | |
setContentView(R.layout.activity_main) | |
db = SQLiteHelperSample(this) | |
val insertBtn = findViewById<Button>(R.id.insert) | |
insertBtn.setOnClickListener { | |
db.insert() | |
} | |
val getBtn = findViewById<Button>(R.id.getAll) | |
getBtn.setOnClickListener { | |
db.getAllData() | |
} | |
val deleteBtn = findViewById<Button>(R.id.deleteAll) | |
deleteBtn.setOnClickListener { | |
db.deleteAll() | |
} | |
} | |
override fun onDestroy() { | |
super.onDestroy() | |
db.close() | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class SQLiteHelperSample(context : Context) : SQLiteOpenHelper(context, DATABASE_NAME, null, DATABASE_VERSION){ | |
companion object { | |
private const val DATABASE_VERSION = 1 | |
private const val DATABASE_NAME = "my.db" | |
private const val TBL_NAME = "my_table" | |
private const val ID = "id" | |
private const val TITLE = "title" | |
private const val CONTENT = "content" | |
} | |
override fun onCreate(db: SQLiteDatabase?) { | |
val SQL_CREATE_ENTRIES = | |
"CREATE TABLE $TBL_NAME (" + | |
"$ID INTEGER PRIMARY KEY," + | |
"$TITLE TEXT," + | |
"$CONTENT TEXT)" | |
db?.execSQL(SQL_CREATE_ENTRIES) | |
} | |
fun insert(){ | |
Log.e("insert", "insert") | |
val db = this.writableDatabase | |
val values = ContentValues().apply { | |
put(TITLE, "TITLE") | |
put(CONTENT, "CONTENT") | |
} | |
db.insert(TBL_NAME, null, values) | |
} | |
fun getAllData(){ | |
Log.e("getAllData", "getAllData") | |
val db = this.readableDatabase | |
val query = "SELECT * FROM $TBL_NAME" | |
val cursor = db.rawQuery(query, null) | |
with(cursor) { | |
while (moveToNext()) { | |
Log.e("this", getInt(0).toString()) | |
Log.e("this", getString(1)) | |
Log.e("this", getString(2)) | |
} | |
} | |
} | |
fun deleteAll(){ | |
Log.e("deleteAll", "deleteAll") | |
val db = this.writableDatabase | |
db.execSQL("DELETE FROM $TBL_NAME") | |
} | |
// Version Upgrade | |
override fun onUpgrade(db: SQLiteDatabase?, oldVersion: Int, newVersion: Int) { | |
db?.execSQL("DROP TABLE IF EXISTS $TBL_NAME") | |
onCreate(db) | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<LinearLayout | |
xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:app="http://schemas.android.com/apk/res-auto" | |
xmlns:tools="http://schemas.android.com/tools" | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
tools:context=".MainActivity"> | |
<Button | |
android:id="@+id/insert" | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:text="insert" | |
/> | |
<Button | |
android:id="@+id/getAll" | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:text="getAll" | |
/> | |
<Button | |
android:id="@+id/deleteAll" | |
android:layout_width="wrap_content" | |
android:layout_height="wrap_content" | |
android:text="delete"/> | |
</LinearLayout> |
- 참조
https://developer.android.com/training/data-storage/sqlite?hl=ko
SQLite를 사용하여 데이터 저장 | Android 개발자 | Android Developers
SQLite를 사용하여 데이터 저장 데이터베이스에 데이터를 저장하는 작업은 연락처 정보와 같이 반복적이거나 구조화된 데이터에 이상적입니다. 이 페이지에서는 개발자가 일반적으로 SQL 데이터
developer.android.com
'Android Jetpack' 카테고리의 다른 글
Android Room - 4 (Room Muti Table / Show DB) (0) | 2022.01.22 |
---|---|
Android Room - 3 (Room Simple ex) (0) | 2022.01.21 |
Android Room -1 (Room vs SQLite) (0) | 2022.01.20 |
Android LiveData - 5 (Livedata Transformations map / switchMap) (0) | 2022.01.15 |
Android LiveData - 4 (Fragment LifeCycleOwner) (0) | 2022.01.09 |