아래와 같이 + 버튼을 누르면 게이지가 올라가는 간단한 예제입니다.
Two Way Binding 과 BindingAdapter를 이용해서 제작해보겠습니다.
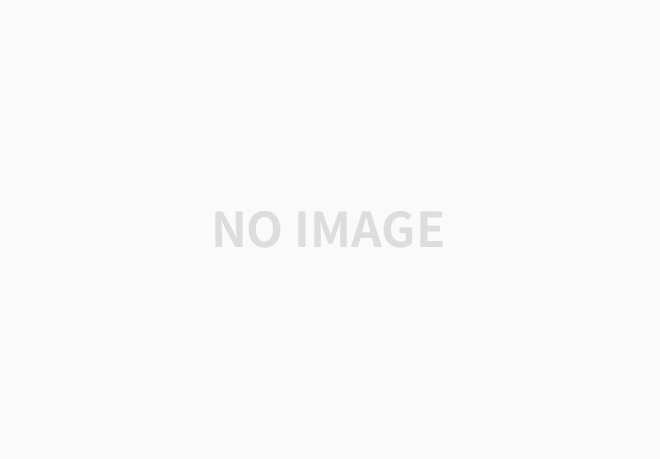
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainActivity : AppCompatActivity() { | |
private lateinit var binding : ActivityMainBinding | |
private lateinit var viewModel: MainViewModel | |
override fun onCreate(savedInstanceState: Bundle?) { | |
super.onCreate(savedInstanceState) | |
binding = DataBindingUtil.setContentView(this, R.layout.activity_main) | |
viewModel = ViewModelProvider(this)[MainViewModel::class.java] | |
binding.vm = viewModel | |
binding.lifecycleOwner = this | |
} | |
} |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainViewModel : ViewModel() { | |
private val _point = MutableLiveData(0) | |
val point : LiveData<Int> = _point | |
val myPointType : LiveData<MyPointType> = Transformations.map(_point){ | |
Log.d("MainViewModel", it.toString()) | |
when { | |
it > 80 -> {MyPointType.BIG} | |
it > 50 -> {MyPointType.MIDDLE} | |
it > 30 -> {MyPointType.SMALL} | |
else -> { MyPointType.VERY_SMALL } | |
} | |
} | |
fun pointPlus(){ | |
_point.value = _point.value?.plus(10) | |
} | |
} | |
enum class MyPointType { | |
BIG, | |
MIDDLE, | |
SMALL, | |
VERY_SMALL | |
} |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
@BindingAdapter("myProgress") | |
fun myProgressSetting(view : ImageView, myPointType: MyPointType) { | |
Log.d("myProgressSetting", myPointType.toString()) | |
when(myPointType) { | |
MyPointType.BIG -> {view.setColorFilter(Color.parseColor("#ff4040"))} | |
MyPointType.MIDDLE -> {view.setColorFilter(Color.parseColor("#fa8072"))} | |
MyPointType.SMALL -> {view.setColorFilter(Color.parseColor("#ffb6c1"))} | |
MyPointType.VERY_SMALL -> {view.setColorFilter(Color.parseColor("#000000"))} | |
} | |
} |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="utf-8"?> | |
<layout | |
xmlns:android="http://schemas.android.com/apk/res/android" | |
xmlns:app="http://schemas.android.com/apk/res-auto" | |
xmlns:tools="http://schemas.android.com/tools"> | |
<data> | |
<variable | |
name="vm" | |
type="com.bokchi.jetpack2_ex.MainViewModel" /> | |
</data> | |
<LinearLayout | |
android:layout_width="match_parent" | |
android:layout_height="match_parent" | |
tools:context=".MainActivity" | |
android:orientation="vertical"> | |
<androidx.constraintlayout.utils.widget.ImageFilterView | |
android:id="@+id/icon" | |
android:src="@drawable/ic_android_black_24dp" | |
myProgress="@{vm.myPointType}" | |
android:layout_width="200dp" | |
android:layout_height="200dp" | |
android:layout_gravity="center"/> | |
<ProgressBar | |
android:id="@+id/progress" | |
android:layout_width="match_parent" | |
android:progress="@{vm.point}" | |
android:layout_height="wrap_content" | |
style="?android:attr/progressBarStyleHorizontal" | |
android:layout_margin="30dp"/> | |
<Button | |
android:id="@+id/plus" | |
android:layout_margin="10dp" | |
android:onClick="@{() -> vm.pointPlus()}" | |
android:text="+" | |
android:layout_width="match_parent" | |
android:layout_height="80dp"/> | |
</LinearLayout> | |
</layout> |
'Android Jetpack' 카테고리의 다른 글
ROOM + Coroutine Flow - 2 (ListAdapter / DiffUtil Ex) (0) | 2023.01.25 |
---|---|
ROOM + Coroutine Flow - 1 (ListAdapter / DiffUtil 이란?) (0) | 2023.01.24 |
DataBinding - 4 (BindingAdapter) (0) | 2023.01.20 |
DataBinding - 3 (BindingAdapter) (0) | 2023.01.20 |
DataBinding - 2 (two - way binding) (0) | 2023.01.16 |