이전 글에서 ViewModel을 쓰지 않고 화면을 뒤집었을 때 계산기 카운터가 초기화 되는 문제를 확인했습니다.
그렇다면, ViewModel을 사용하면 이 문제를 어떻게 해결할 수 있을까요?
ViewModel의 사용법
그러면 ViewModel을 이용해서 한번 살펴보겠습니다.
MainViewModel 을 만들어서 변수 countValue를 선언해줍니다.
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainViewModel : ViewModel() { | |
var countValue = 0 | |
} |
그런 후에, Activity에서 viewModel을 아래와 같은 코드로 연결해줍니다.
lateinit var viewModel: MainViewModel
viewModel = ViewModelProvider(this).get(MainViewModel::class.java)
전체 Activity 코드를 보면 아래와 같습니다.
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainActivity : AppCompatActivity() { | |
lateinit var binding : ActivityMainBinding | |
lateinit var viewModel: MainViewModel | |
override fun onCreate(savedInstanceState: Bundle?) { | |
super.onCreate(savedInstanceState) | |
binding = DataBindingUtil.setContentView(this, R.layout.activity_main) | |
viewModel = ViewModelProvider(this).get(MainViewModel::class.java) | |
binding.countShowArea.text = viewModel.countValue.toString() | |
binding.plusBtn.setOnClickListener { | |
viewModel.countValue++ | |
binding.countShowArea.text = viewModel.countValue.toString() | |
} | |
binding.minusBtn.setOnClickListener { | |
viewModel.countValue-- | |
binding.countShowArea.text = viewModel.countValue.toString() | |
} | |
} | |
} |
다시 ViewModel 데이터를 활용해서 계산기를 회전시키면 아래와 같이 데이터가 유지되는 것을 볼 수 있습니다~
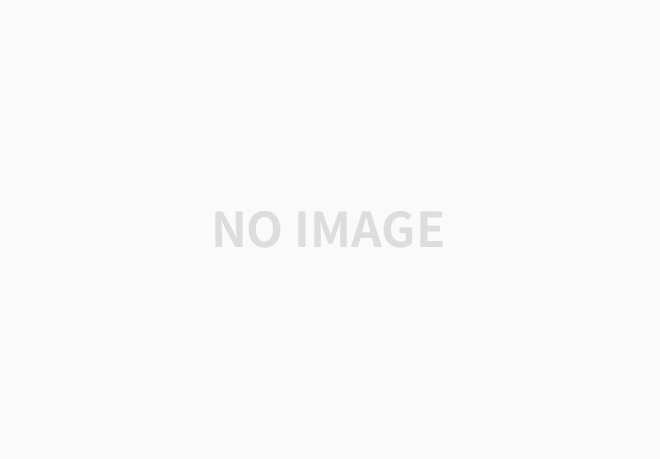
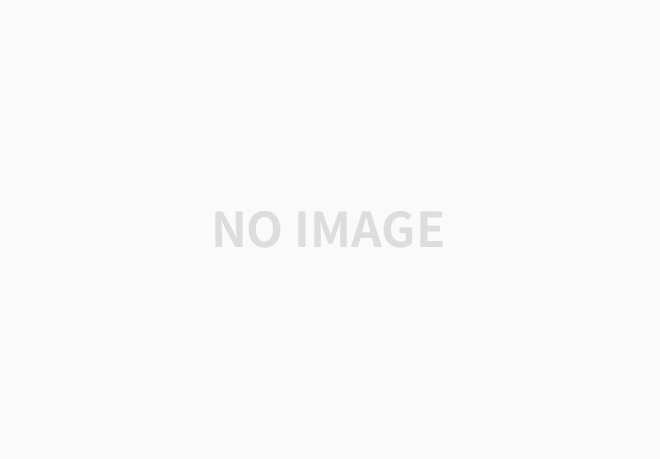
추후, LiveData / MVVM 패턴 등에서도 많이 활용되니, 잘 알아두시면 좋습니다 :)
-- 추가
위의 코드보다 아래의 코드를 참고하시면 좋습니다.
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainActivity : AppCompatActivity() { | |
lateinit var viewModel : MainViewModel | |
override fun onCreate(savedInstanceState: Bundle?) { | |
super.onCreate(savedInstanceState) | |
setContentView(R.layout.activity_main) | |
viewModel = ViewModelProvider(this).get(MainViewModel::class.java) | |
Log.d("MainActivity", "onCreate") | |
val plusBtn : Button = findViewById(R.id.plus) | |
val minusBtn : Button = findViewById(R.id.minus) | |
val resultArea : TextView = findViewById(R.id.result) | |
resultArea.text = viewModel.countValue.toString() | |
plusBtn.setOnClickListener { | |
viewModel.plus() | |
Log.d("MainActivity", viewModel.getCount().toString()) | |
resultArea.text = viewModel.getCount().toString() | |
} | |
minusBtn.setOnClickListener { | |
viewModel.minus() | |
resultArea.text = viewModel.getCount().toString() | |
} | |
} | |
override fun onStart() { | |
super.onStart() | |
Log.d("MainActivity", "onStart") | |
} | |
override fun onResume() { | |
super.onResume() | |
Log.d("MainActivity", "onResume") | |
} | |
override fun onPause() { | |
super.onPause() | |
Log.d("MainActivity", "onPause") | |
} | |
override fun onStop() { | |
super.onStop() | |
Log.d("MainActivity", "onStop") | |
} | |
override fun onDestroy() { | |
super.onDestroy() | |
Log.d("MainActivity", "onDestroy") | |
} | |
} |
This file contains hidden or bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainViewModel : ViewModel() { | |
var countValue : Int = 0 | |
init { | |
Log.d("MainViewModel", "init") | |
} | |
fun plus(){ | |
countValue++ | |
} | |
fun minus(){ | |
countValue-- | |
} | |
fun getCount(): Int { | |
return countValue | |
} | |
} |
- 참조
https://medium.com/androiddevelopers/viewmodels-a-simple-example-ed5ac416317e
'Android Jetpack' 카테고리의 다른 글
Android ViewModel - 4 (Activity Fragment ViewModel 공유) (0) | 2021.12.22 |
---|---|
Android ViewModel - 3 (Fragment에서 사용) (0) | 2021.12.18 |
Android ViewModel - 1 (ViewModel의 필요성) (0) | 2021.12.18 |
Android View에 대한 접근 - 4 (Databinding with DataClass) (0) | 2021.12.15 |
Android View에 대한 접근 - 3 (databinding) (0) | 2021.12.14 |